Step 1: Open Xcode select the windows based application template give your project an appropriate name, after doing this add UIViewController subclass file to your application. In iPhone if we want to draw some shapes or perform any sort of drawing stuff then in that case you have to take a separate view and then add this view to your view controller class, you may follow this mechanism not only for drawing geometric views but to create your custom views like a login control, etc. So now after adding UIViewController subclass file add UIView subclass file and name it Rectangle, since it’s the rectangle shape that we will be drawing first,
Step 2: Select the Rectangle.m file and inside that file you will find a method called as drawRect, the drawRect method allows the programmer to create his/her own custom views, so in this case I just want a small rectangle to appear in my view so for that add the following code in the drawRect method
- (void)drawRect:(CGRect)rect {
// Drawing code
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSetLineWidth(context, 3.0f);
CGContextSetStrokeColorWithColor(context, [UIColor blueColor].CGColor);
CGContextAddRect(context,CGRectMake(80, 80, 120, 120));
CGContextStrokePath(context);
}
Code Explanation:
CGContextRef : It represents a drawing environment where the developer can draw his views. The drawRect method takes a parameter called as rect and this method gets called directly, so now when you will create the object of this method in the myviewController.m file and pass the parameter of frames to the init method of the rectangle class this frame will be referred to as the current context and inside the current context you can draw anything
UIGraphicsCurrentContext(): It represents the current graphics context.
CGContextSetStrokeColorWithColor: This method will set the color for the context
CGContextAddRect: This method will add a rectangle path in the current context, it takes two parameter one is the context in which you want to draw the rectangle and the other is the frame of the rectangle like its height, width, x and y.
CGContextStrokePath: This method will paint a line along the given path in the context.
Step 3: Select the myviewController.h file and declare the object of the rectangle class
#import
#import "Rectangle.h"
@interface myviewController : UIViewController {
Rectangle *r;
}
@end
and now traverse to the myviewController.m file and select the loadView method and add this piece of code
- (void)loadView {
[super loadView];
r = [[Rectangle alloc]initWithFrame:CGRectMake(20, 82, 280, 348)];
[self.view addSubview:r];
}
Code Explanation: The above code just adds the rectangle view to the current view.
Step 4: Select the appDelegate.m file and add the myviewController view to the iPhone window
#import "DrawingShapesAppDelegate.h"
#import "myviewController.h"
@implementation DrawingShapesAppDelegate
@synthesize window;
- (void)applicationDidFinishLaunching:(UIApplication *)application {
// Override point for customization after application launch
myviewController *obj = [[myviewController alloc]init];
[window addSubview:obj.view];
[window makeKeyAndVisible];
}
Step 5: Press build and go to get the output just like below
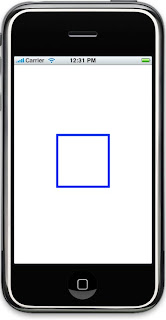
- (void)drawRect:(CGRect)rect {
// Drawing code
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSetLineWidth(context, 3.0f);
CGContextAddRect(context,CGRectMake(80, 80, 120, 120));
CGContextSetFillColorWithColor(context, [UIColor blueColor].CGColor);
CGContextFillPath(context);
}